C Advanced
Categoria Corso
Development & Programming, ICTech
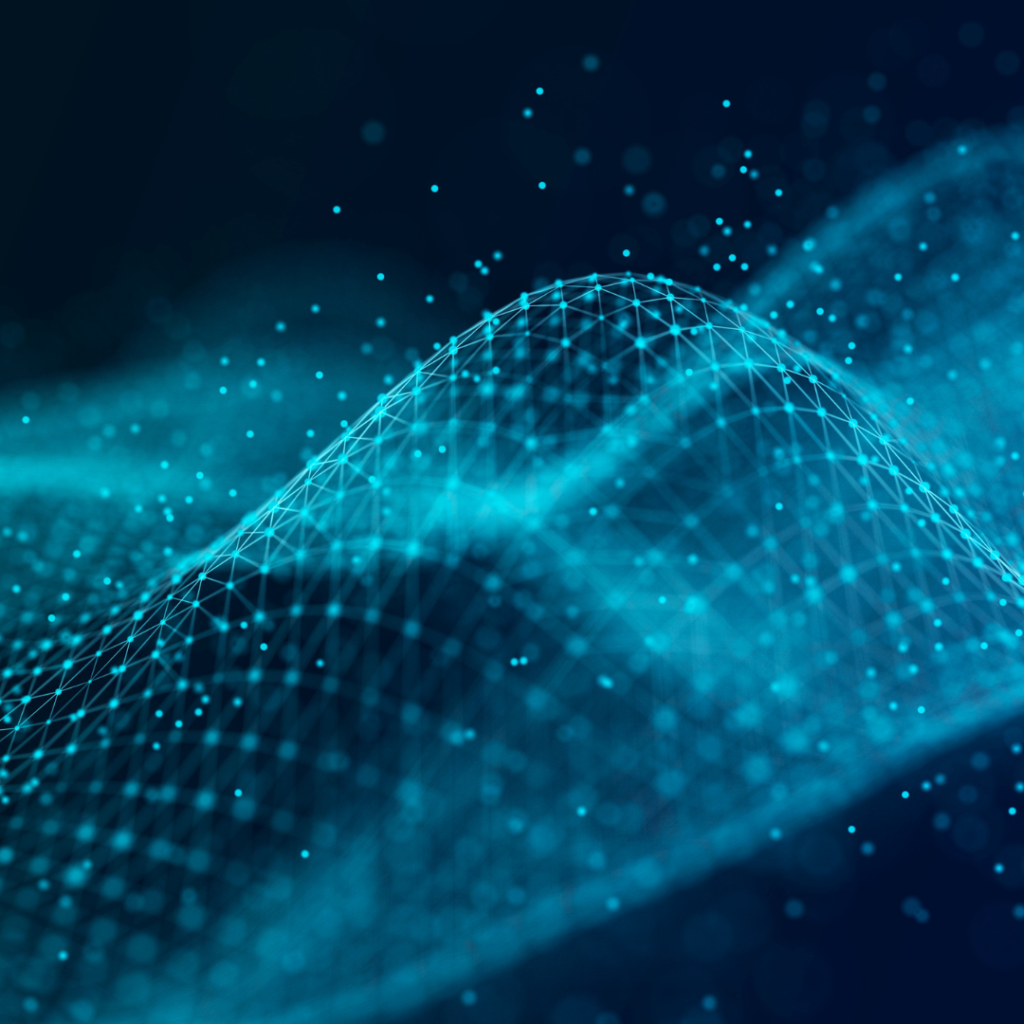
CLP 12-01 – C++ Institute
2-4 giorni
Virtual ClassRoom
In Presenza
Development & Programming, ICTech
Corso avanzato di programmazione in C, sviluppato con C++ Institute, aiuta ad acquisire conoscenza della sintassi e della semantica del linguaggio C, nonché tipi di dati avanzati come librerie, concetti di programmazione e strumenti di sviluppo, capacità di identificare bug e colli di bottiglia di codice, programmazione di strutture dati avanzate, risoluzione di problemi non banali con l’utilizzo di strutture dati e algoritmi, progettazione e scrittura di programmi mediante infrastrutture linguistiche standard
Il corso prepara alla certificazione CLP 12-01.
Evolution of C – from past to eternity
Handling variable number of parameters (<stdarg.h>)
Low level IO (<unistd.h>)
Memory and strings (<string.h> et al.)
Processes and threads
Floats and ints once again (<math.h>, <fenv.h>, <inttypes.h> et al.)
Network sockets – absolute basics
Miscellaneous
Aver frequentato il corso C Essentials o essere in possesso analoghe competenze
Prepara all’esame di certificazione CLP 12-01 del C++ Institute da prenotare e pagare a parte
Maggiori informazioni su: CLP – C Certified Professional Programmer
Attestato ITHUM di partecipazione al corso
Ricevi aggiornamenti su novità e corsi di formazione
ITHUM S.r.l.
Via Cristoforo Colombo, 149
00147 – Roma
Amministrazione (+39) 06 86726329
Formazione (+39) 06 2158915
ithum@pec.ithum.it
informazioni@ithum.it
P.IVA 08493511003
INNOVAZIONE – RICERCA – CONDIVISIONE
Insieme alla crescita della persona sono i valori che guidano ogni nostro passo nel percorso di Formazione e Consulenza
Azienda certificata ISO 9001